I’ve managed to create an interactive plot that contains several series. When I click on one of a glyph on one serie, a histogram plot is updated and print the forces associated with this glyph.
To walk you through the code, I start by uploading a csv that all contain loads of data with the same column names. Then the code reads the csv and filters it with different widgets and only plot the filtered data. So I end up with a graph with different lines corresponding to each filtered csv. Because the function I’ve created to create the histogram doesn’t know which serie I’m clicking on, I’ve worked around this by adding the forces I want to plot to the data of the column data source of each serie. I store them like you store hover points.
And everything works like a charm except when I’m adding the forces in the column data source. To do this, I filter each serie and take only the column that contains ‘Fx [N]’, ‘Fy [N]’, etc… and then add it to the source.
This used to work well but for some reason, sometimes nothing shows on the graph even if I can easily print the data and bokeh has no problem reading and finding it. So nothing shows up on the graph and I can’t click on any point to show the forces after.
Bokeh doesn’t print any error.
The first code is a function called whenever I filter the data with one of the widget. It updates the data source and plots the new line. (I’ll put a screenshot of what it looks like below) If I remove the ‘fx’, ‘fy’, etc in the source_p1[j].data, it works again but I can’t plot the histogram.
def update_p1_serie(given_serie, j):
p1.xaxis.axis_label = x_p1.value
p1.yaxis.axis_label = y_p1.value
if not given_serie.empty:
given_serie.sort_values(by=[x_p1.value], inplace=True)
xs_p1 = given_serie[x_p1.value].values
ys_p1 = given_serie[y_p1.value].values
forces_data_fx = given_serie.filter(like='Fx (N)')
forces_data_fy = given_serie.filter(like='Fy (N)')
forces_data_fz = given_serie.filter(like='Fz (N)')
forces_data_mx = given_serie.filter(like='Mx (N.m)')
forces_data_my = given_serie.filter(like='My (N.m)')
forces_data_mz = given_serie.filter(like='Mz (N.m)')
source_p1[j].data = dict(x=xs_p1, y=ys_p1, hover102=given_serie[para102],
hover103=given_serie[para103], hover104=given_serie[para104],
hover105=given_serie[para105], hover1=given_serie[para1],
hover2=given_serie[name_para2], hover3=given_serie[name_para3],
hover4=given_serie[para4], hover5=given_serie[para5],
hover6=given_serie[para6], hover7=given_serie[para7],
hover8=given_serie[para8], hover9=given_serie[para9],
hover10=given_serie[para10], hover11=given_serie[para11], fx=forces_data_fx,
fy=forces_data_fy, fz=forces_data_fz, mx=forces_data_mx, my=forces_data_my,
mz=forces_data_mz)
p1.line(x='x', y='y', source=source_p1[j], line_color=colors[j], line_width=2, alpha=1,
name=series_name[j])
r1 = p1.circle(x='x', y='y', source=source_p1[j], color=colors[j], size=6, alpha=1, hover_alpha=1,
name=series_name[j])
else:
source_p1[j].data = dict(x=[], y=[])
This second code is the function called when I click on a glyph and that plots the histogram. It shouldn’t be the problem since it’s not even called before the problem. (I only show the example for ‘fx’ since I do the same for the other forces)
def update_bar(event):
SELECTED = [None] * (len(source_p1))
for j, source in enumerate(source_p1):
SELECTED[j] = source_p1[j].selected.indices
if len(SELECTED[j]) > 1:
SELECTED[j] = SELECTED[j][-1]
if SELECTED[j]:
values_fx = []
values_fy = []
values_fz = []
values_mx = []
values_my = []
values_mz = []
for i, column in enumerate(source_p1[j].data['fx'].columns.tolist()):
values_fx.append(source_p1[j].data['fx'][column][SELECTED[j]].values.tolist())
values_fx = [x[0] for x in values_fx]
data_dict_fx = {'column': forces_name_fx, 'top': values_fx}
source_bar_fx.data = data_dict_fx
bar_fx.hbar(y='column', right='top', width=0.9, source=source_bar_fx,
line_color='black', color=colors[j])
bar_fx.xgrid.grid_line_color = None
bar_fx.add_tools(HoverTool(tooltips=[('Série', series_name[j]), ('Modèle', "@column"), ('Valeur', '@top')]))
The first photo is when everything works, I’ve clicked on a point and it prints the forces associated with it. The second one is after I started filtered a bit more or just changing certain values, it starts glitching like that. I’ve also noticed that it usually shows something on the graph when I haven’t yet filtered the data except when the csv file has less than 9 lines which is even stranger.
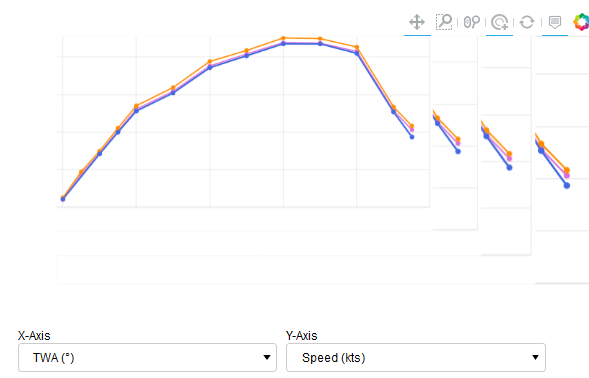
Is there something I’m missing ? Or is there a different solution I should be using that could do the same thing ?
Hopefully I was clear enough, let me know if you need more info to understand the problem. I can’t unfortunately put the entire code as it’s confidential and pretty long.
Any help would be greatly appreciated.