I have created a sample code. Please go through below code and guide me how I will get something similar to above image mention early in this post.
from bokeh.io import curdoc
from bokeh.plotting import figure, output_file, show
from bokeh.models import FreehandDrawTool, ColumnDataSource, Segment, CustomJS, Line, MultiLine
from bokeh.plotting import figure, output_file, show
from bokeh.models import PolyDrawTool, PolyEditTool
p = figure(x_range=(0, 10), y_range=(0, 10), width=400, height=400,
title='Poly Edit Tool')
l1 = p.multi_line([], [], line_width =5, alpha= 0.4, color='red', line_dash = 'dashed')
draw_tool_l1 = PolyDrawTool(renderers=[l1], num_objects=3)
source2= ColumnDataSource(data=dict(x=[], y=[], x1= [], y1= []))
source = ColumnDataSource(data=dict(x=[], y=[], x1= [], y1= []))
callback = CustomJS(args=dict(x_range = p.x_range,y_range = p.y_range,source=source, source2 = source2), code="""
// the event that triggered the callback is cb_obj:
// The event type determines the relevant attributes
console.log('Tap event occurred at x-position: ' + cb_obj.x)
console.log('Tap event occurred at y-position: ' + cb_obj.y)
//var data = source.data;
x0 = cb_obj.x;
y0 = cb_obj.y;
x30 = (x0*0.3) + x0;
y30 = (x0*0.3) + y0;
source.data.x.push(x0);
source.data.y.push(y0);
source2.data.x30.push(x30);
source2.data.y30.push(y30);
source.data.y1.push(y0);
source2.data.y1.push(y0);
source2.data.x1.push(y_range.max);
source.data.x1.push(y_range.max);
console.log('x-position: ' + source2.data.x30);
console.log('1x-position: ' + source.data.x);
console.log('y-position: ' + source2.data.y30);
console.log('x1-position: ' + source2.data.x1);
console.log('y1-position: ' + source2.data.y1);
source.change.emit();
""")
# execute a callback whenever the plot canvas is tapped
p.js_on_event('doubletap', callback)
s = Segment(x0='x',y0='y',x1='x1',y1='y1')
s1 = Segment(x0='x',y0='y',x1='x1',y1='y1')
s2 = Segment(x0='x30',y0='y30',x1='x1',y1='y1')
p.add_glyph(source, s, selection_glyph=s, nonselection_glyph=s)
p.add_glyph(source, s1, selection_glyph=s1, nonselection_glyph=s1)
p.add_glyph(source2, s2, selection_glyph=s2, nonselection_glyph=s2)
p.add_tools(draw_tool_l1)
p.toolbar.active_drag = draw_tool_l1
curdoc().add_root(p)
Output of given code , I am not getting extra segment “s2”, which uses “source2” CDS.
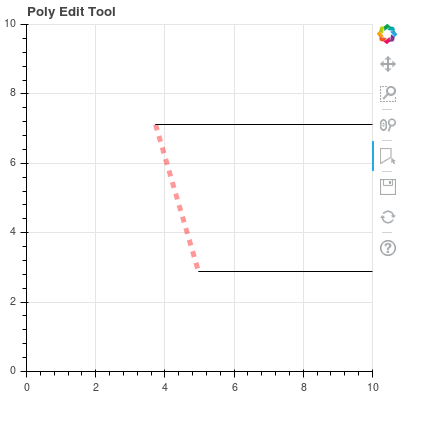
Thank You.